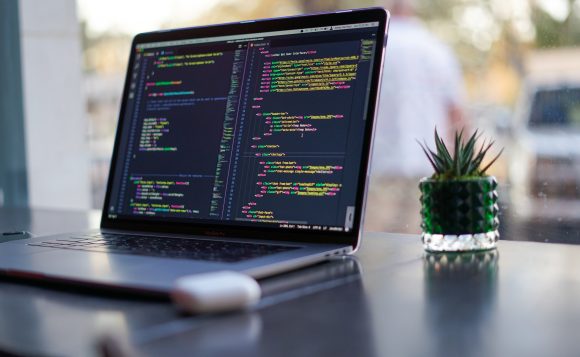
Sometimes we get requests for using native java methods from Unity code in the Oculus Application. Below, we describe one of the ways we can check if Wi-Fi and internet connection are enabled on the Oculus headset from the Unity Engine code part.
In this sample application, we check if Wi-Fi is switched on or off on the Oculus app and if we have an internet connection to this Wi-Fi.
At first, we need to call the native java class method using this code to call Wi-Fi availability:
///
/// Send request to JavaClass to check if wifi is enabled on oculus
///
///
private bool DeviceWiFiAndroidRequest ()
{
var isWifiEnabled = false;
try
{
// An activity provides the window where the app draws its UI.
// Typically, one activity in an app is specified as the main activity, which is the first screen to appear when the user launches the app.
// Get current activity in unity project from java classes.
// AndroidJavaClass: Construct an AndroidJavaClass from the class name.
// GetStatic: Get the value of a static field in an object type.
using (var activity =
new AndroidJavaClass ("com.unity3d.player.UnityPlayer").
GetStatic ("currentActivity"))
{
// Method "getSystemService": Gets the name of the system-level service that is represented by the specified class.
// As the parameter arguments, we pass the name of the package with handle "getSystemService".
// The class of the returned object varies by the requested name.
// Get "WifiManager" for management of Wi-Fi connectivity.
using (var wifiManager = activity.Call ("getSystemService", "wifi"))
{
// Call: Calls a Java method on an object. Call, T - return type
// Call method "isWifiEnabled". Return whether Wi-Fi is enabled or disabled. Returns bool value.
isWifiEnabled = wifiManager.Call ("isWifiEnabled");
}
}
}
catch (Exception e)
{
Debug.LogError ("Android request exception: " + e.Message);
}
return isWifiEnabled;
}
After that, we need to check if we have an internet connection on connected Wi-Fi. Also, we need to call the native java class method.
///
/// Request to JavaClass to check if internet connection is reachable
///
///
private bool NetworkConnectionAndroidRequest ()
{
var isNetworkEnabled = false;
try
{
using (var activity =
new AndroidJavaClass ("com.unity3d.player.UnityPlayer").
GetStatic ("currentActivity"))
{
// Get "ConnectivityManager" for handling management of network connections.
using (var connectivityManager =
activity.Call ("getSystemService", "connectivity"))
{
// Call method "getActiveNetworkInfo" that returns details about the currently active default data network.
// Call method "isConnected" that indicates if network connectivity exists. Returns bool value.
isNetworkEnabled =
connectivityManager.Call ("getActiveNetworkInfo").
Call ("isConnected");
}
}
}
catch (Exception e)
{
Debug.LogError ("Android request exception: " + e.Message);
}
return isNetworkEnabled;
}
After this, we have to use a coroutine to check these two parameters. We check each for 2 seconds.
///
/// Check if the application is connected to Wi-Fi in coroutine
///
///
private IEnumerator CheckDeviceWifiConnection ()
{
yield return new WaitForSecondsRealtime (2f);
while (true)
{
yield return new WaitForSecondsRealtime (checkDelay);
wifiConnection = DeviceWiFiAndroidRequest ();
wifiMessage.text = wifiConnection ? "Wifi On" : "Wifi Off";
}
}
///
/// Check if the application is connected to Wi-Fi in coroutine
///
///
private IEnumerator CheckNetworkConnection ()
{
yield return new WaitForSecondsRealtime (2f);
while (true)
{
yield return new WaitForSecondsRealtime (checkDelay);
internetConnection = NetworkConnectionAndroidRequest ();
internetMessage.text =
internetConnection ? "Internet reachable" :
"Internet not reachable";
}
}
Below, you can see the full script for checking internet connection and Wi-Fi availability on the Oculus Quest 2 from Unity code using native java methods.